Coding 101: If/Else Statements Simplified
- Test Lead
- Sep 6, 2023
- 3 min read
This article will try to simplify the concept of If/Else statements in computer science programming. An if/else statement is an example of a conditional statement. If a certain condition is met, then an action will take place. You use if/else statements every day in your life without realizing it. See the below flowchart as an example.
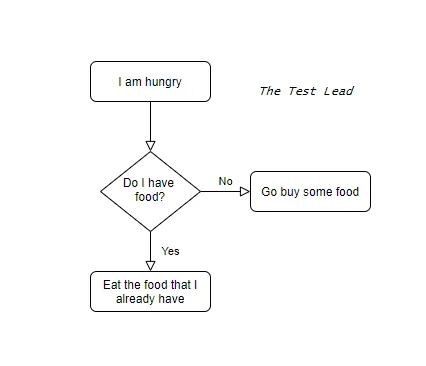
The current situation is that you are hungry. Next, we will see if you have food. If you do have food, you will just eat the food you already have. Else, you will go buy some food to eat. This is a basic example of an if statement.
In computer science, just like in real life as seen in our flowchart, in an if statement, if a certain condition is met then a specific action will take place. If that condition is not met then that action will not take place and another action may take place instead.
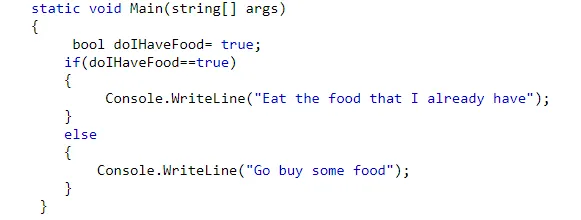
The above line of code demonstrates what was earlier shown in our flowchart. We know that we are hungry. Our if statement checks if we have food. Because “doIHaveFood” is equal to true then this block of code will print “Eat the food that I already have”. If “doIHaveFood” was equal to false, the code would print out “Go buy some food”
Comparison Operators
If you noticed in our if statement, we had “==” in between the 2 values that we wanted to compare. This is an example of our comparison operators. Comparison operators are used to check if a statement is true or not. Use the comparison operator that best matches your needs. Look at the list below of the other comparison operators along with their meanings
Comparison Operators
== equal to (left side is equal to the right side)
> greater than (left side is greater than the right side)
≥ greater than or equal to (left side is greater than or equal to the right side)
≤ less than or equal to (left side is less than or equal to the right side)
!= not equal to (left side is not equal to the right side)
Logical Operators
Next, we have logical operators. The main 2 logical operators are AND and OR. AND is represented by &&, OR is represented by ||. When using the &&(AND) both conditions must be met. When using the ||(OR) only 1 of the conditions have to be met.
With the first 2 screenshots, you see when both conditions are met, “I can buy food” is printed. However, in screenshot 2 when 1 condition is not true, “I need to get a job” is printed.
And Operator
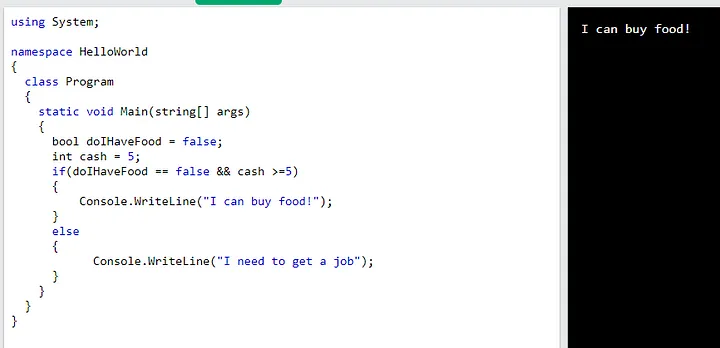
Failing AND Operator
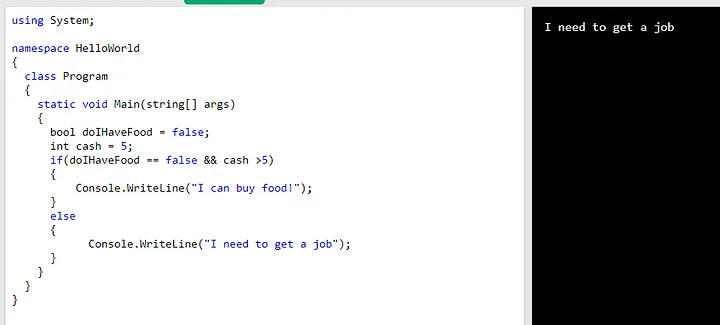
In the final screenshot below you can see the OR operator. As long as either I did not have food or cash was more than 5, “I can buy food” is printed
OR Operator
ElseIf
Now we will finish we “If Else”. Think of this as a way to be more precise with your information and give more options. Imagine you have $20 and go to the store and want to know what you could buy. You go up to the price tag of the TV first and see it’s $500, which is more than you have so you go to the next item. After that, you go to the video games. A new Xbox game is $60, which is still more than you have so you can’t get that either. Finally, you find a nice book for $19.99, so you get it. However, because you spent all of your money on the book, you didn’t get to buy the soda that was $2.
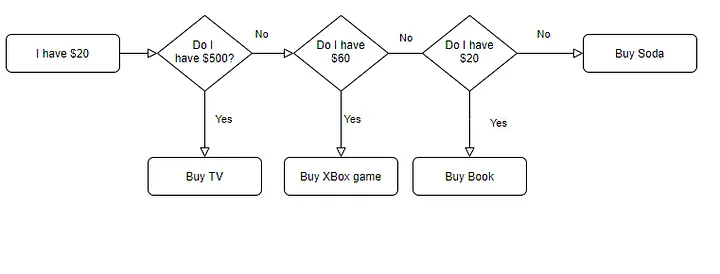
The ElseIf goes into each elseIf statement until one of the criteria is met. Once the criteria are met then it executes the code for that block and then ignores the following elseif statements. See the below code snippet.
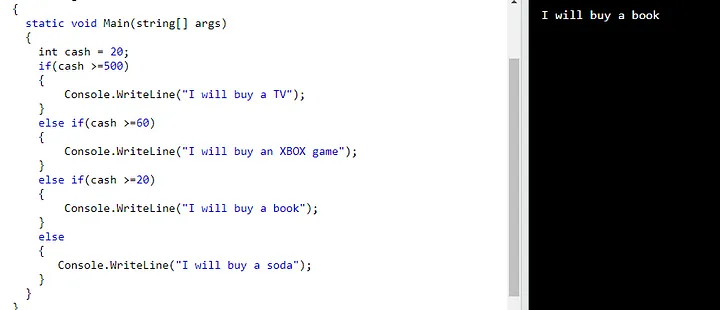
Comments